Frescospangríe
Creativity is intelligence having fun.
Frescospangríe
The work of art presented before us is a captivating visual exploration of the interplay between the organic and the abstract. The piece, entitled "Frescospangríe," (pronounced ‘fres-co-span-gree-ay’) is composed of numerous intersecting lines and dots of varying sizes and vivid colors, arranged in a seemingly chaotic manner. This unique composition is a product of a randomly generated color palette, as well as a programmatic selection of coordinates that results in a composition of randomly placed lines and dots.
Each line of the composition is carefully selected using a specific algorithm that ensures that none of the lines intersect. This algorithm also serves to ensure that the dots are not placed inside of any of the lines, resulting in an aesthetically pleasing piece. The bright and vibrant colors that adorn the composition further add to the captivating nature of the piece, drawing the eye in to explore its intricate details.
Ultimately, this piece serves as a testament to the potential for artistic expression that can be achieved through the use of carefully crafted algorithms. By leveraging the power of programming, the artist is able to create a work of art that is both visually striking and technically complex. The resulting piece is a captivating exploration of the interplay between the organic and the abstract, and is a truly unique experience that is sure to leave viewers in awe.
Some examples of what this code creates
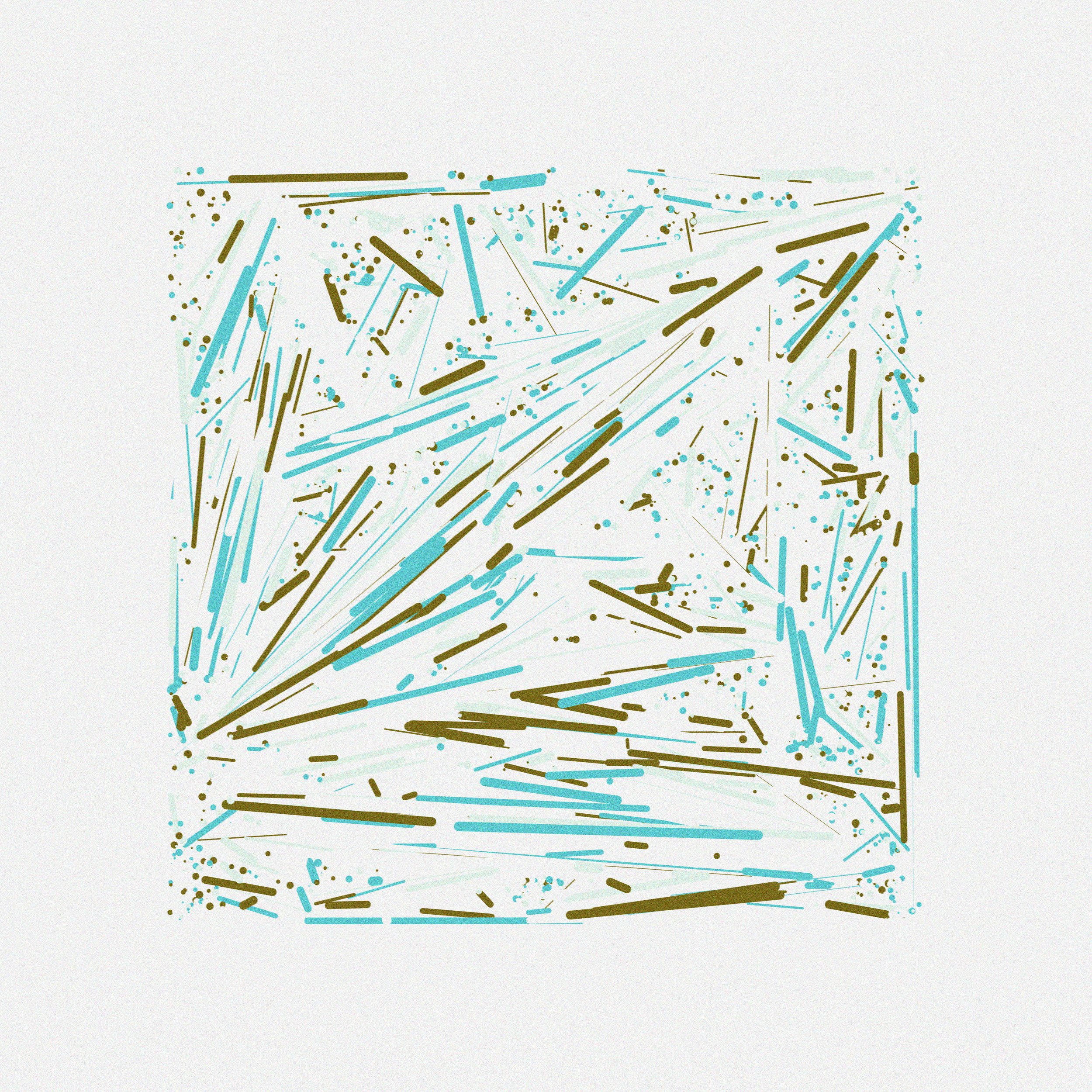
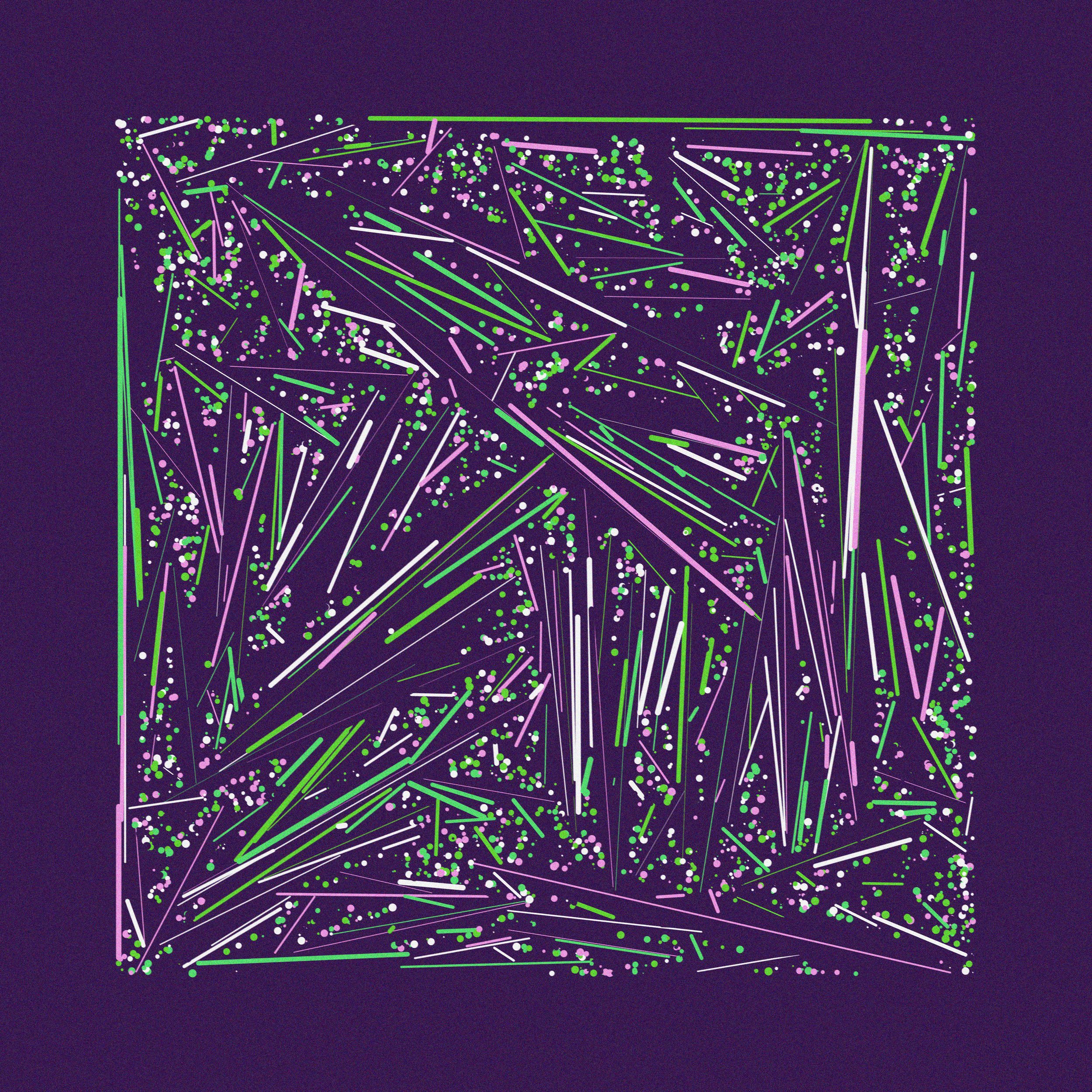
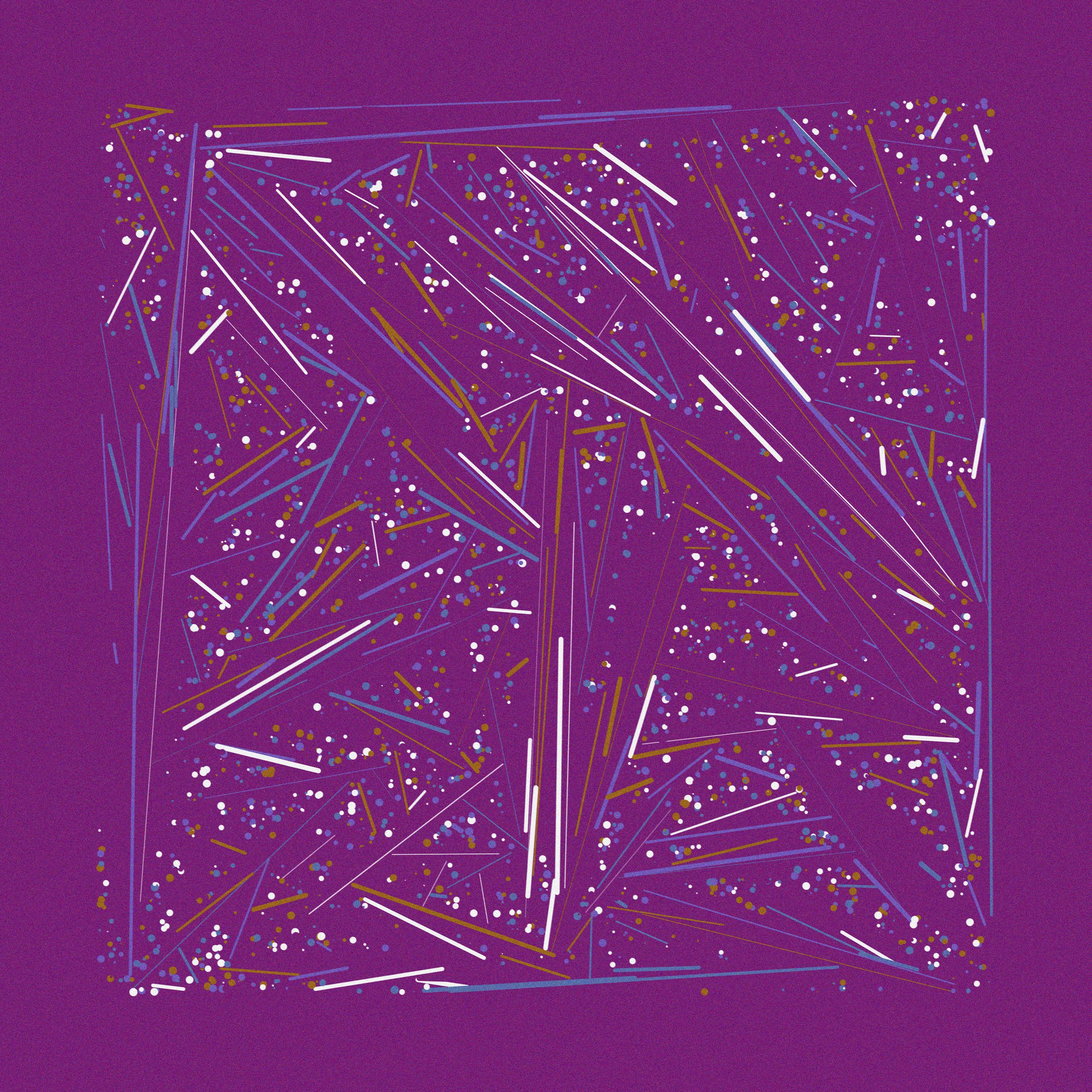
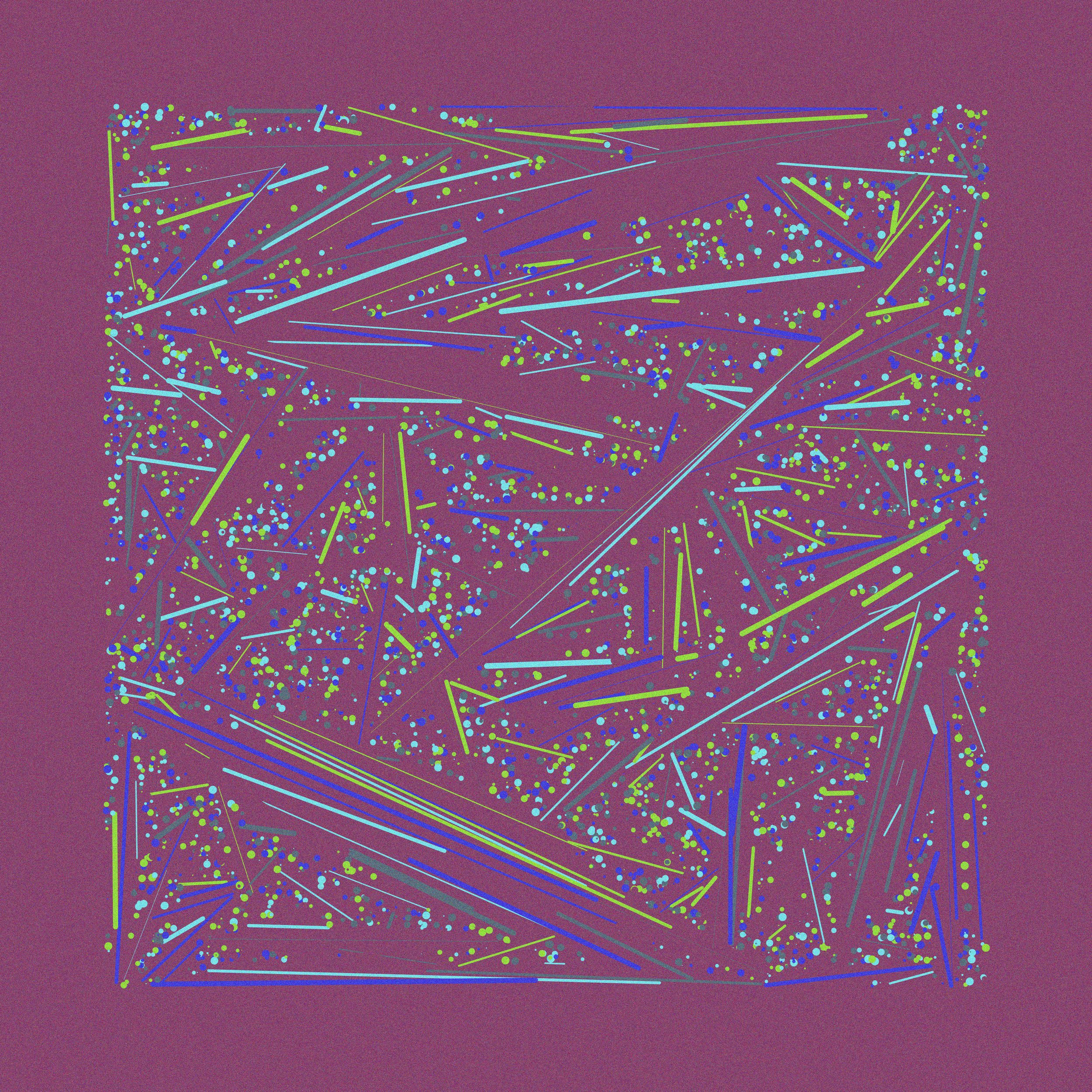
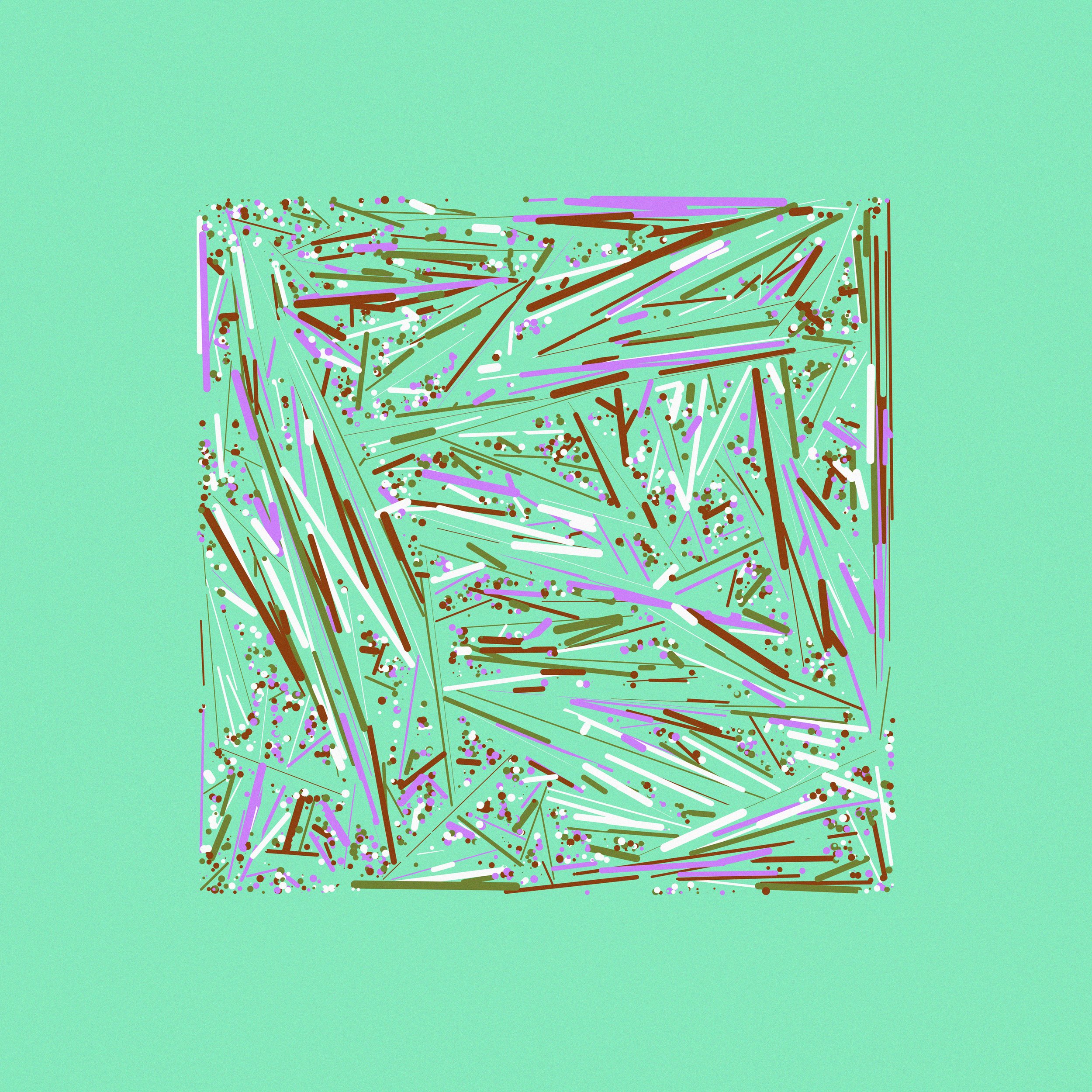
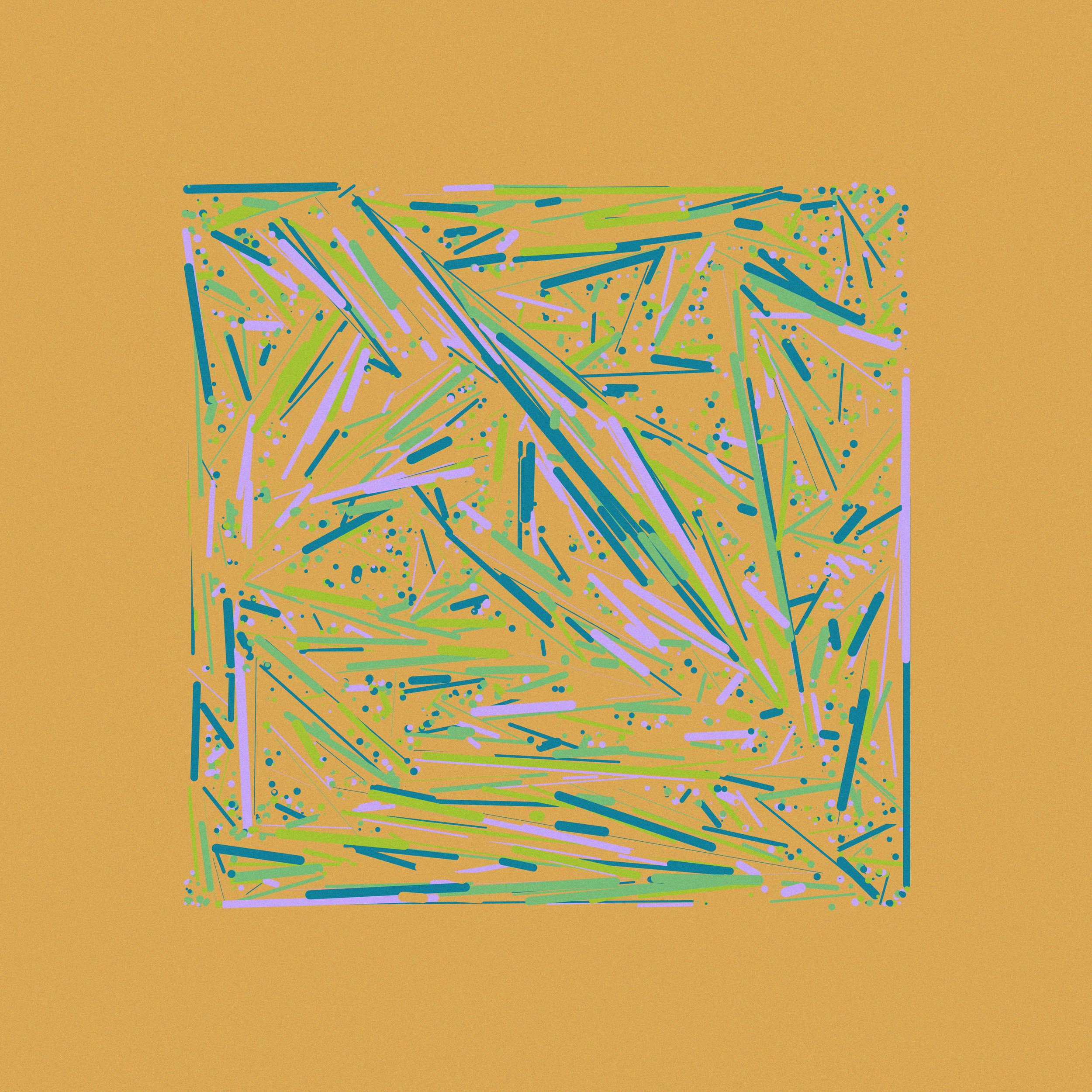
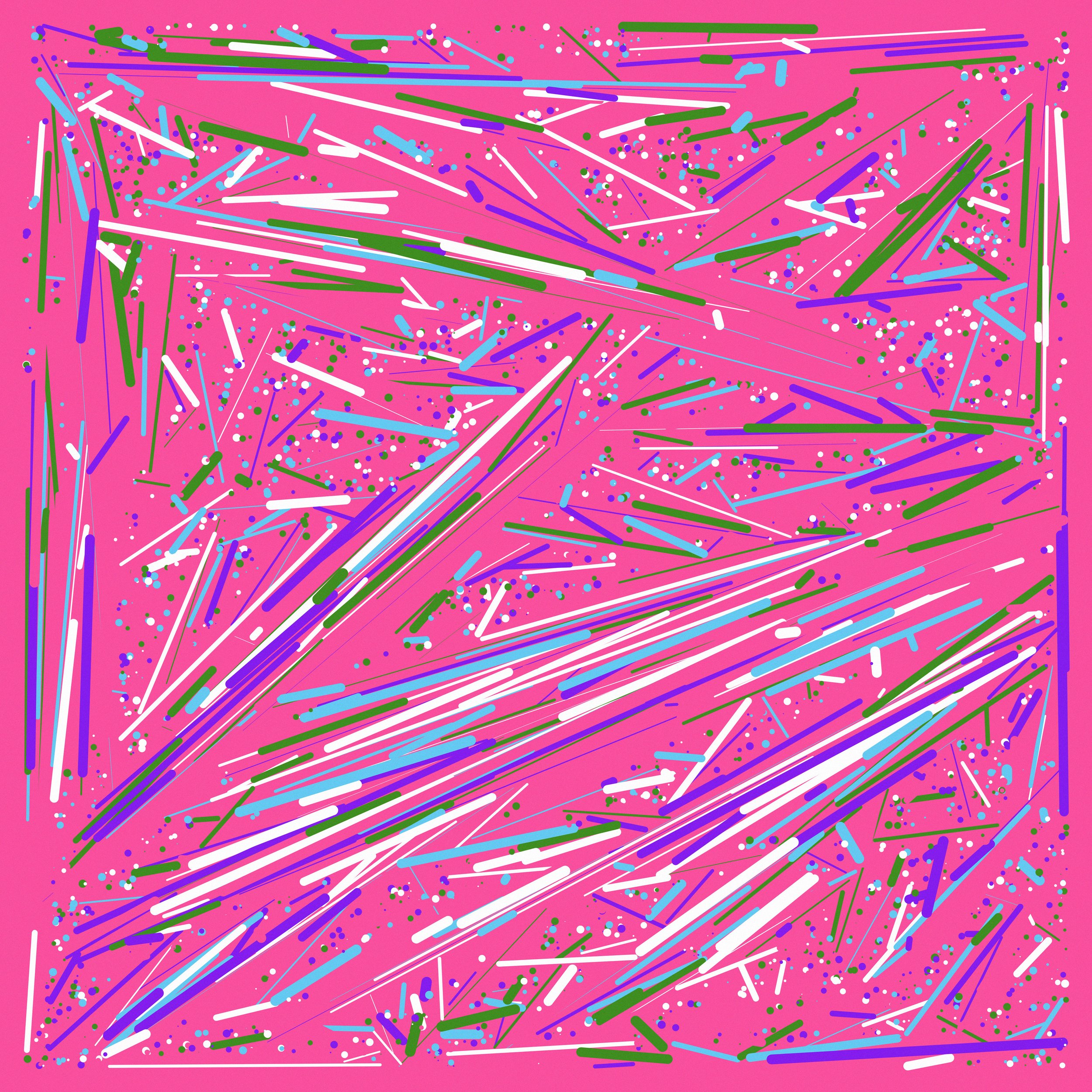
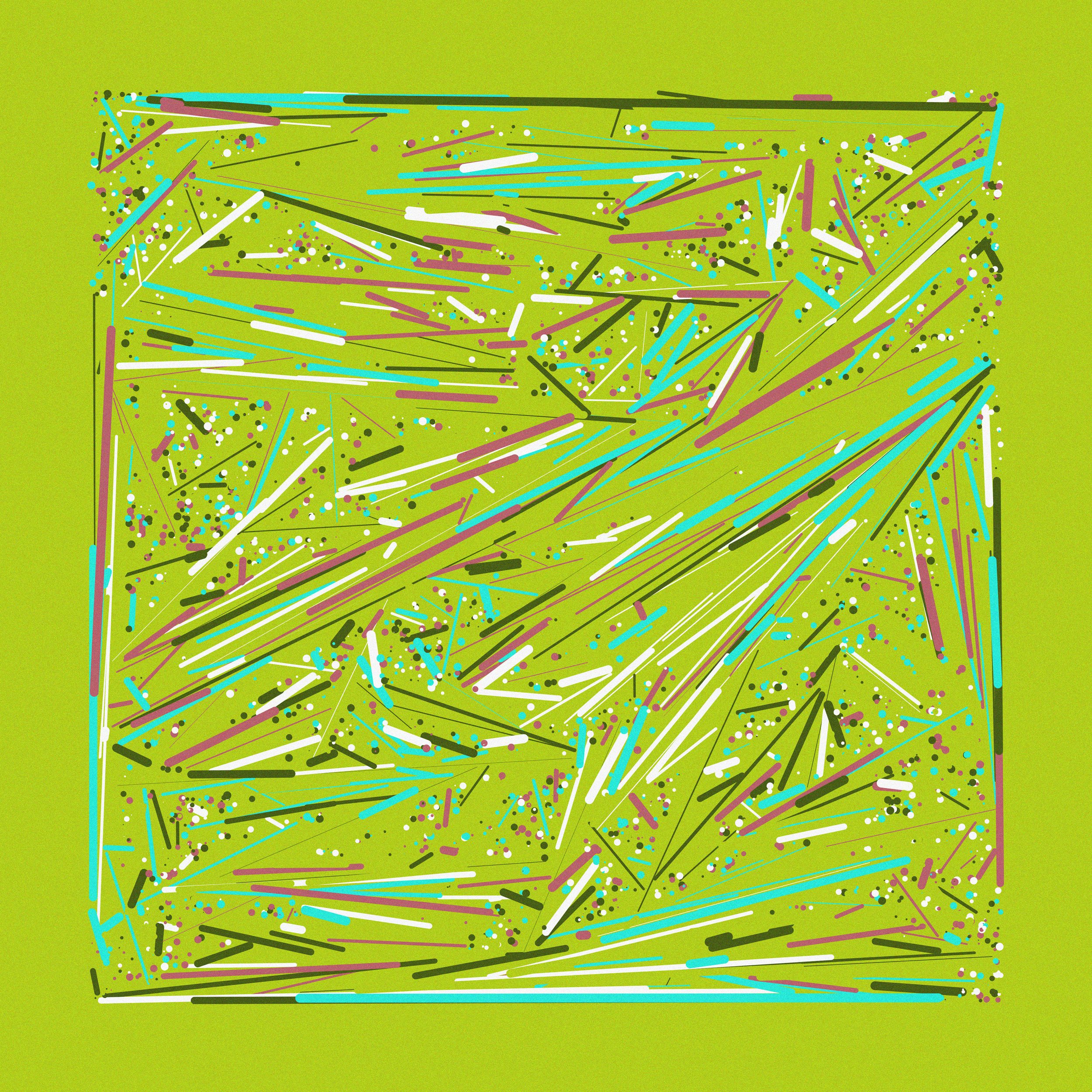
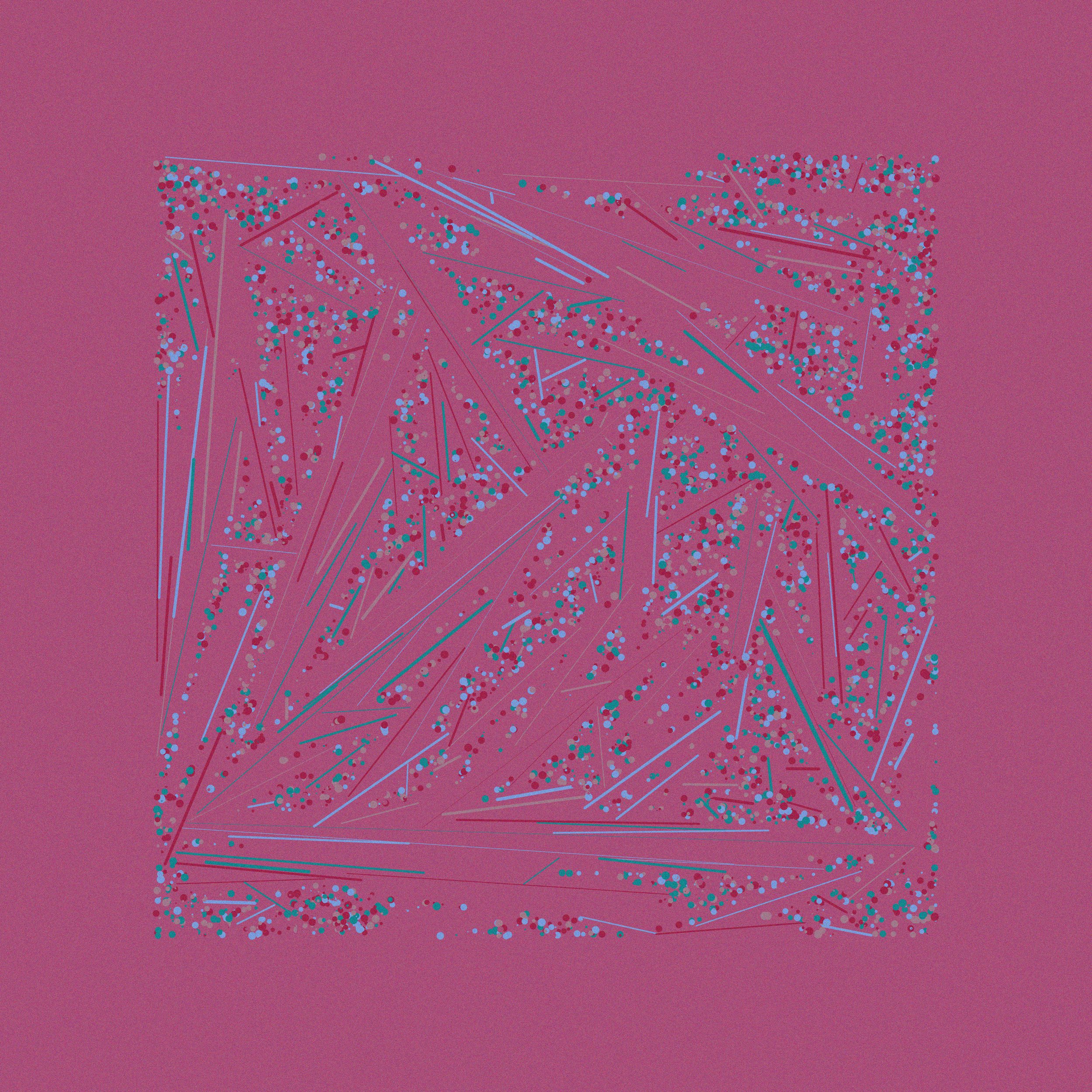
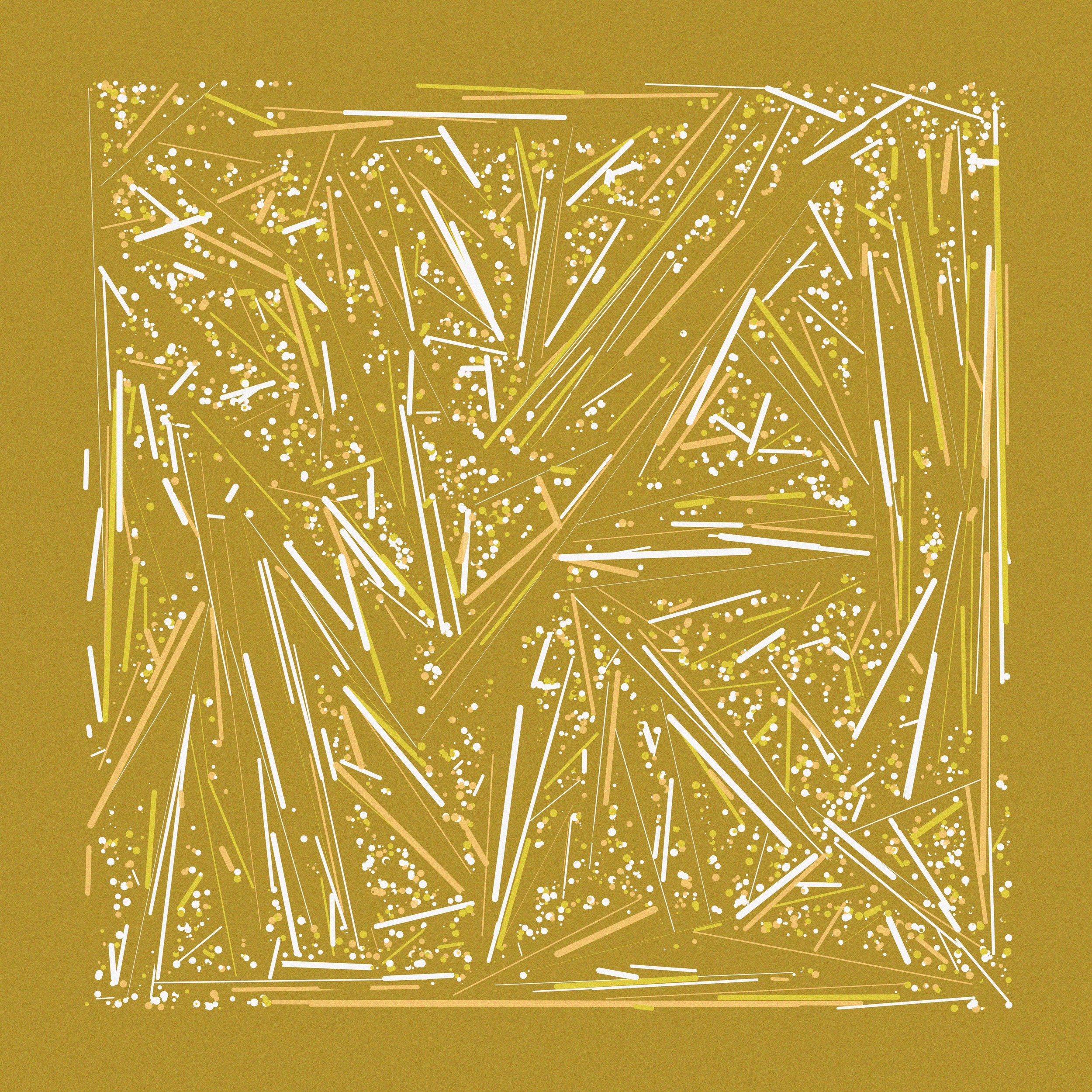
Frescospangríe Code:
//
// ______ __
// | ____| /_/
// | |__ _ __ ___ ___ ___ ___ ___ _ __ __ _ _ __ __ _ _ __ _ ___
// | __| '__/ _ \/ __|/ __/ _ \/ __| '_ \ / _` | '_ \ / _` | '__| |/ _ \
// | | | | | __/\__ \ (_| (_) \__ \ |_) | (_| | | | | (_| | | | | __/
// |_| |_| \___||___/\___\___/|___/ .__/ \__,_|_| |_|\__, |_| |_|\___|
// | | __/ |
// |_| |___/
//
function setup() {
createCanvas(4000, 4000);
noLoop();
}
// GranulateFuzzify code from:
// https://www.fxhash.xyz/article/all-about-that-grain
function granulateFuzzify(amount) {
loadPixels();
const d = pixelDensity();
const fuzzyPixels = random(0,3); // pixels
const modC = 4 * fuzzyPixels; // channels * pixels
const modW = 4 * width * d;
const pixelsCount = modW * (height * d);
for (let i = 0; i < pixelsCount; i += 4) {
const f = modC + modW;
// fuzzify
if (pixels[i+f]) {
pixels[i] = round((pixels[i] + pixels[i+f])/2);
pixels[i+1] = round((pixels[i+1] + pixels[i+f+1])/2);
pixels[i+2] = round((pixels[i+2] + pixels[i+f+2])/2);
}
// granulate
pixels[i] = pixels[i] + random(-amount, amount);
pixels[i+1] = pixels[i+1] + random(-amount, amount);
pixels[i+2] = pixels[i+2] + random(-amount, amount);
}
updatePixels();
}
// Save a PNG when 'S' key is pressed.
function keyPressed() {
if (key === "s" || key === "S") {
saveCanvas("Frescospangríe", "png");
}
}
function draw() {
// Number of lines and dots drawn
let max = 100000;
let min = 10000;
// Set margin between 0 and 750
let margin = random(0, 750);
// randomly generate a color palette
let colorPalette = [];
for (let i = 0; i < 5; i++) {
let r = int(random(0, 256));
let g = int(random(0, 256));
let b = int(random(0, 256));
let color = "#" + r.toString(16) + g.toString(16) + b.toString(16);
colorPalette.push(color);
}
// Generate random background color from palette.
background(colorPalette[0]);
// Draw Lines
let numLines = int(random(min, max));
let lineWeight = 1;
let lines = [];
for (let i = 0; i < numLines; i++) {
let x1 = random(margin, width - margin);
let y1 = random(margin, height - margin);
let x2 = random(margin, width - margin);
let y2 = random(margin, height - margin);
let linePos = [x1, y1, x2, y2];
// check if the line intersects with any other lines
let validLine = true;
for (let j = 0; j < lines.length; j++) {
let lineA = lines[j];
if (doIntersect(lineA, linePos)) {
validLine = false;
}
}
// if the line doesn't intersect, draw it and add it to the array of lines
if (validLine) {
strokeWeight(lineWeight);
stroke(colorPalette[int(random(0, colorPalette.length))]);
line(x1, y1, x2, y2);
lineWeight += 0.05;
lines.push(linePos);
}
}
// add dots
let numDots = int(random(10000, 20000));
for (let i = 0; i < numDots; i++) {
let x = random(margin, width - margin);
let y = random(margin, height - margin);
// check if the dot is inside a line
let validDot = true;
for (let j = 0; j < lines.length; j++) {
let lineA = lines[j];
if (isInLine(lineA, x, y)) {
validDot = false;
}
}
// if the dot isn't in a line, draw it
if (validDot) {
strokeWeight(random(2, 30));
stroke(colorPalette[int(random(0, colorPalette.length))]);
point(x, y);
}
}
// checks if two lines intersect
function doIntersect(lineA, lineB) {
let x1 = lineA[0];
let y1 = lineA[1];
let x2 = lineA[2];
let y2 = lineA[3];
let x3 = lineB[0];
let y3 = lineB[1];
let x4 = lineB[2];
let y4 = lineB[3];
let denominator = (y4 - y3) * (x2 - x1) - (x4 - x3) * (y2 - y1);
if (denominator == 0) {
return false;
}
let uA = ((x4 - x3) * (y1 - y3) - (y4 - y3) * (x1 - x3)) / denominator;
let uB = ((x2 - x1) * (y1 - y3) - (y2 - y1) * (x1 - x3)) / denominator;
if (uA >= 0 && uA <= 1 && uB >= 0 && uB <= 1) {
return true;
}
return false;
}
// checks if a point is inside a line
function isInLine(lineA, x, y) {
let x1 = lineA[0];
let y1 = lineA[1];
let x2 = lineA[2];
let y2 = lineA[3];
let d = dist(x, y, x1, y1) + dist(x, y, x2, y2);
let lineLength = dist(x1, y1, x2, y2);
if (d >= lineLength - 5 && d <= lineLength + 5) {
return true;
}
return false;
}
granulateFuzzify(random(5,35));
}